Git is a widely adopted version control system that was originally developed by Linus Torvalds in 2005 and has since been maintained by Junio Hamano. It is well-known for its ability to track code changes, monitor contributors who made those changes, and enable effective coding collaboration.
Git is a powerful tool that allows you to manage your projects by creating repositories, cloning them to your local machine to work on, controlling and tracking changes with staging and committing, branching and merging to enable you to work on different parts and versions of a project, pulling the latest version of the project to your local machine, and pushing your local updates back to the main project.
When working with Git, you can initialize it on a folder to create a repository, after which Git will create a hidden folder to track changes in that folder. Any changes made to the files in that folder, such as modifications, additions or deletions, are then considered modified. You can then select the modified files you want to stage and commit, which prompts Git to store a permanent snapshot of those files. Git also allows you to view the full history of every commit and revert back to any previous commit if needed. Notably, Git keeps track of the changes made in each commit, rather than storing a separate copy of every file in every commit.
Installation
- Ubuntu
- # sudo apt install git
- # git --version
- Windows
- Download and Install from here.
Configure
- # git config --global user.name "github-username"
- # git config --global user.email "github-email"
Pushing code to GitHub using Git.
To learn Git and GitHub, a simple project is an excellent starting point that allows you to cover the required concepts in action. For instance, let's consider a project in IoT that involves both software and hardware components, with three remote team members collaborating on the project. Assuming you are the team lead, you have already created the project structure and assigned tasks to other team members. Now, the question arises, how do you share the code you have created?
Before you can share the code, you need to take care of version control for this project, and the most widely used version control system for software development is Git. However, to use Git in your project, you need to set it up first. Therefore, the first step is to initialize Git on the project folder, which creates a Git repository that tracks changes in the project. Then, you can add and commit your initial code changes to Git, creating a new branch for each new feature or functionality added to the project.
Create a project folder first.
# mkdir newProject
Go to the project directory# cd newProject/
Check if there are any files present by using this command:
# ls -a
Initialise the git repository.
# git init
Now again, check if there are any files present by using this command:
# ls -a
Git creates a hidden folder to keep track of changes (.git).
Git can track any changes made within the directory, and you can view these changes using a specific command. Thus, to observe any changes occurring in the directory, utilize this command.
# git status
Since there have been no changes made, you won't be able to see any modifications. So, proceed to create your project structure, and then reattempt the command to observe any changes.
# git status
After creating three files, Git is now tracking the changes made, and you can observe them.
Now the next step is staging the changes. Staging refers to the process of selecting and preparing changes that you want to include in your next commit. When you make changes to your files in a Git repository, Git recognises these changes but does not automatically commit them. Instead, you must explicitly tell Git which changes to include in your next commit by staging them using the git add command. This adds the changes to the staging area, where they are ready to be committed.
# git add --all
Committing refers to the process of permanently saving the changes in the repository. Once you have staged your changes, you can commit them using the git commit command. This creates a new commit in the Git repository that records the changes you made. You can also add a commit message to describe the changes you made, which can be useful for yourself and other collaborators who may need to review the changes.
# git commit -m "Initial commit"
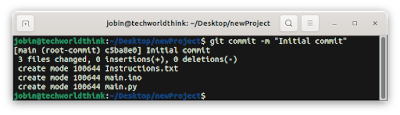
After tracking and committing the changes, we are now ready to move on to GitHub. Start by creating an account on GitHub and then proceed to create a repository. Once your repository is set up on GitHub, you will need to establish a connection with your local repository by setting up the origin. To do this, copy the remote repository URL and use the below command to set up the origin within your local repository.
# git remote add origin <remote repository URL>
You can now upload your code changes to the repository by using the command below:
# git push -u origin main
This command will transfer your local changes to the remote repository on GitHub, allowing you to share your work with others and collaborate effectively. So, feel free to push your changes to the repository and let Git handle the rest for you.
Comments
Post a Comment